Project Symmetry: Devblog 3
As the year draws to a close, I thought it might be a good idea to squeeze in one more blog post just to document the current state the project is in. I haven’t been able to work on the project as much as I wanted. I’ve only been able to work intermittently here and there but things are ramping up!
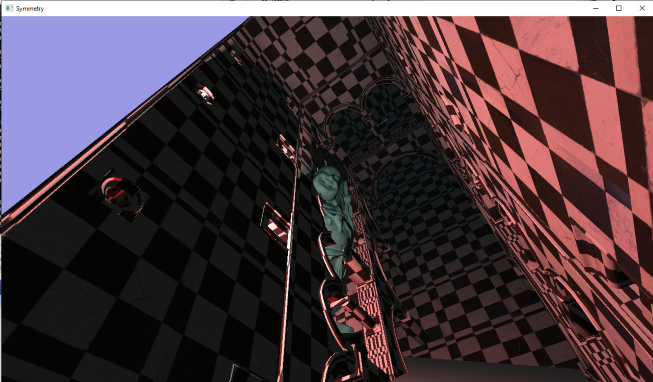
I’ve been making a lot of progress this past month on the serialization related aspects. Things that relate to writing the state of the game into files and re-creating the state from those files again. I can now create my own entities and save them in a simple format. Here’s an example:
Entity
{
type : 6
material : 0
diffuse_color : 1.000 1.000 1.000 1.000
geometry : sponza.symbres
specular : 1.0000
active : true
diffuse_texture : default.tga
diffuse : 1.0000
specular_strength : 1.0000
name : Sponza
}
Its quite barebones and thats really the point. It serves as a simple way for me to define entity archetypes that I can then instantiate into the scene and either use as is, or to use a base and then add modifications on top and then save it along with the scene. When this entity is saved in a scene file it is differentiated from a normal entity by saving it as a “Scene_Entity_Entry” instead of being saved as a normal entity. This signals that this entity is to be loaded from another file and then the rest of the changes are applied on top. In a scene file, it looks like this:
Scene_Entity_Entry
{
scale : 3.000 3.000 3.000
rotation : 0.000 0.000 0.000 1.000
position : -13.000 1.000 1.000
filename : Sponza
name : Sponza
}
So while this doesn’t really support anything fancy like having the ability to nest archetypes inside one another, it does support other child entities. What this means is that I can essentially save any part of the scene hierarchy into a file and then load it as is or instanitate it whenever I want and create variations by building on it which can be really helpful.
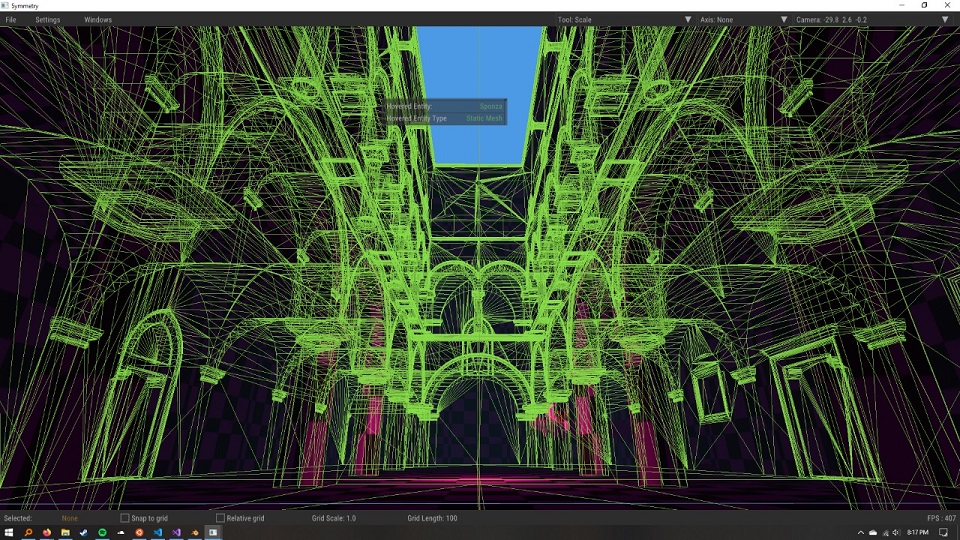
The scene file itself follow a similar simple format. In the beginning we have an entry for the scene related data itself called “Scene_Config” which specifies the configuration of global scene data like the color of the ambient light and so on. This is again is somewhat barebones at the moment but I wanted something simple that I could easily add to in the future.
Although the player is an entity just like any other, we handle the player entity differently. For example, each scene will always have a player entity and the basic structure is defined in the code. I feel that constraints like these help me from making something overly generic that I may or may not need in the future and keep me focused on what I need. I can always add something when I need but if I keep writing solutions for problems I’ll never encounter then I’m only wasting time. A principle more widely known as YAGNI.
This is then followed by all the entities in the scene that are either added programmatically or through the editor itself. Here how it might actually look like when we have a scene with only the player and a light entity called “Test_Light” in it:
Scene_Config
{
debug_draw_color : 0.800 0.400 0.100 1.000
fog_type : 1
fog_density : 0.1000
fog_color : 0.170 0.490 0.630
debug_draw_physics : false
fog_start_distance : 10.0000
fog_max_distance : 450.0000
debug_draw_enabled : false
debug_draw_mode : 0
ambient_light : 0.100 0.100 0.100
}
Player
{
type : 2
scale : 1.000 1.000 1.000
rotation : 0.000 0.771 0.000 0.636
active : true
position : 21.479 5.660 -3.077
name : Player
camera_clear_color : 0.600 0.600 0.900 1.000
}
Entity
{
type : 5
scale : 1.000 1.000 1.000
inner_angle : 20.0000
falloff : 1.5000
light_type : 2
depth_bias : 0.0005
rotation : 0.000 0.000 0.000 1.000
cast_shadow : false
intensity : 1.0000
color : 1.000 1.000 1.000
active : true
radius : 20.0000
position : 0.000 5.000 0.000
outer_angle : 30.0000
name : Test_Light
pcf_enabled : false
valid : true
}
I feel like I’m at a very good position now where the editor is almost ready to make some simple test levels. I just need to make a few more additions in how scenes are loaded and unloaded and we’ll be to pump out some basic levels for the game.
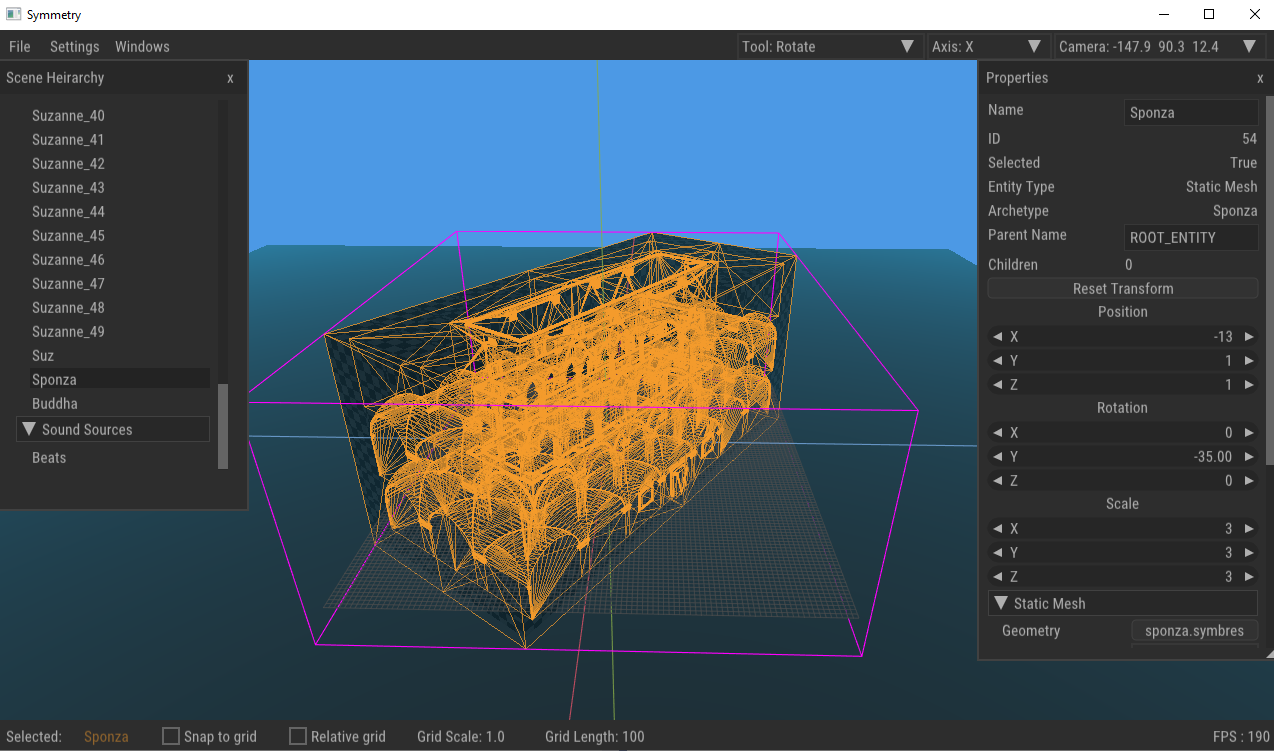
I’ve been yearning to get into more gameplay side of things because even though going down these rabbit holes is fun, its what I’ve been doing for a very long time. To be able to make something from scratch and have other people download it on their machines and be able to play it is going to be its own kind of reward and I can’t wait to get there!
Project Source Code : Github.